MERN Full Stack Developer Tutorial
The MERN stack is a group of tools that help JavaScript developers create dependable and expandable web apps. As this skill is increasingly in demand, we came up with our MERN Full-Stack Developer tutorial, which gives you a fundamental understanding of every technology.
Introduction to MERN Full Stack
MongoDB, Express, React, and Node.js are the components that make up the acronym “MERN,” and each one has a specific function in the development process.
- MongoDB: MongoDB is a document-oriented database that is effective at storing JSON-formatted data.
- Express.JS: Express is a framework for online applications that offers several capabilities to make the process of putting together web apps easier.
- React.JS: The JavaScript front-end package React provides a comprehensive toolkit for creating user interfaces.
- Node.JS: The runtime environment used to coordinate communication between front-end and back-end components when JavaScript code is executed on the server side is called Node.js.
Use Cases of MERN Stack
Applications that are efficient and easy to use are emerging from the MERN stack, such as the following:
Real-time application handling: The “N” in MERN stands for Node.js, which is perfect for managing real-time data streams in websites with chat, gaming, and collaboration tools.
Single page applications (SPAs): Web applications known as SPAs load a single HTML page and allow for dynamic updates without requiring a page reload.
The “R” in MERN, or React, a well-known collection of JavaScript components that makes it simple to create updateable SPAs like social media pages, web email services, and maps, activates this capability.
Data-driven applications: The letter “M” in MERN stands for MongoDB, a NoSQL database for storing and retrieving enormous amounts of data in document format.
The “E” in MERN, Express, is a very effective framework for controlling communication between the database and the front end. Content management systems, social networking apps, and e-commerce apps are a few examples of data-driven applications.
In this tutorial, we cover the following:
- Understanding of front-end technologies: HTML, CSS, JavaScript, and Bootstrap.
- Understanding of back-end Technologies: Node.Js, React.Js, and Express.Js
- Understanding of databases: MySQL & MongoDB
Understanding of Front End Technologies: HTML, CSS, JavaScript, and Bootstrap
Front-end web development includes features that contribute to the responsiveness, interactivity, and aesthetic appeal of websites.
The primary components of front-end web development, HTML, CSS, and JavaScript, are combined to create the user experience.
HTML: Foundation Blocks
A website’s structure is composed of HTML or HyperText Markup Language.
- By giving the content distinct properties, you can use HTML to add diverse components to the website design. This contributes to the layout of the webpage.
- HTML is made up of tags that define how a certain piece of material will look and behave. Tags can be used to bold or italicize text, link to a picture, and more.
- These tags can be added before and after the required content to create headlines, lists, links, paragraphs, and other elements. They are contained in angle brackets.
- HTML further codes properties like a class that assists target a specific set of elements and provide secret information about the element.
CSS: Design and Style
After the website’s HTML layout is complete, you may incorporate CSS, or Cascading Style Sheets, to enhance each element’s or section’s look. Color, typefaces, layout, spacing, and content animation are all included in this.
The rulesets utilized by this design language consist of:
Selector: This establishes the HTML components that should be positioned and styled at the beginning of the ruleset.
Declaration: This is the style, such as color, font, or spacing, that you would like to alter for that specific element. It is further separated into:
- Property: It chooses the specific kind of attribute that should be created for the element.
- Property Value: It alludes to how the element might look, including the font, color, and padding.
JavaScript: Activity and Interaction
By enabling elements to react in a certain way when viewed, JavaScript contributes to the interactivity of your webpage.
- You can use JavaScript to develop a responsive user interface that includes element interactions like dropdown menus, animations, and form validation.
- To do this, one has to have access to the Document Object Model (DOM), a programming interface that shows the hierarchy of a webpage.
This API allows you to modify various aspects as needed. JavaScript has the following features:
- Variables: In your script, you can declare a variable and give it an array, boolean, string, number, or object value.
- Comments: You can add these short texts to the code for your reference.
- Operators: These are mathematical symbols that represent the interaction of two variables.
- Conditionals: They assist in determining if an expression is true or false.
- Functions: You can assign a function to several elements at once with these commands, saving you from having to write the same code twice.
- Events: Events are code structures that detect browser activity and execute the necessary code to make the website interactive.
Understanding of back-end Technologies: Node.Js, React.Js, and Express.Js
We must establish a front-end and back-end folder structure to set up the MERN stack. The database structure must then be defined to store and retrieve data from the database.
Step 1: Make a new project folder after installing the code editor. Next, navigate to the project folder in the terminal or command prompt and enter the following instructions to create frontend and backend folders.
mkdir frontend
mkdir backend
Step 2: Use the command to navigate to the frontend folder.
cd frontend
Step 3: Use the command to launch a React project
npx create-react-app
Step 4: Now use the command to browse to the backend folder.
cd..
cd backend
Step 5: Use the command to initialize the project’s backend.
npm init -y
Step 6: Use the command to install Express and other backend dependencies
npm i mongodb express cors dotenv
MERN Full Stack Components: Explained
When utilizing the MERN stack, developers employ React, Express, and Node to implement the website’s view layer, and MongoDB is used to implement the database layer.
Express.JS
Express is a framework for Node.js. Excellent web apps and APIs can be designed with the aid of Express. Because Express offers so many middlewares, writing code is simplified and made shorter.
Benefits of Express.JS
- Single-threaded and asynchronous.
- Quick, easy, and expandable
- It has the largest Node community.
- Because js Express has an integrated router, it encourages code reuse.
- Robust API
Step 1: To begin your Express project, create a new folder and use the following command to initialize a package at the command prompt.JSON data set. Proceed after accepting the default options.
npm init
Step 2: Next, use the command below to install Express, then press Enter. At last, produce a file called index.js within the directory.
npm install express –save
Step 3: In index.js, type the following to build an example server.
const express=require(‘express’),
http=require(‘http’);
const hostname=’localhost’;
const port=8080;
const app=express();
app.use((req, res)=> {
console.log(req.headers);
res.statusCode=200;
res.setHeader(‘Content-Type’, ‘text/html’);
res.end(‘<html><body><h1>This is a test server</h1></body></html>’);
});
const sample_server=http.createServer(app);
sample_server.listen(port, hostname, ()=> {
console.log(`Server running at http: //${hostname}:${port}/`);});
Step 4: Update the package.json file’s “scripts” section.
Step 5: Use the command below to launch the server.
npm start
Step 6: The output of the server is now visible when you open a browser.
React.JS
A JavaScript package called React is used to create user interfaces. React’s ability to handle rapidly changing data makes it a popular choice for developing single-page and mobile applications. With React, users may write JavaScript code and design user interface elements.
Benefits of React.JS
Below are the advantages of React.JS:
Virtual DOM: A virtual DOM object is a DOM object’s representation.
JSX: The acronym for JavaScript XML is JSX. React uses this JavaScript extension for HTML and XML.
Components: The fundamental units of a user interface are its components, each of which has a purpose and adds to the overall design.
High Performance: Compared to other frameworks, it operates significantly faster due to features like virtual DOM, JSX, and components.
Creating Android and iOS Apps: React Native makes it simple to create Android and iOS apps using JavaScript and ReactJS.
Step 1: Before you can launch your React application, use npm or yarn to install “create-react-app.”
npm install create-react-app –global
Or
yarn global add create-react-app
Step 2: Following that, you may use React to create a new application.
create-react-app app_name
Step 3: Next, open the “app_name” folder and launch your application by typing yarn start or npm start.
Step 4: This is how a typical React application looks:
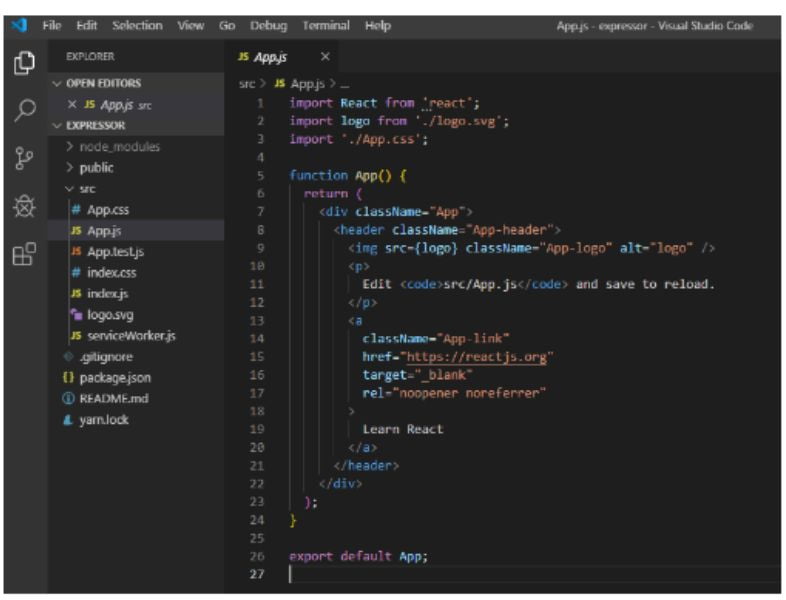
Step 5: Modify the index.js file
ReactDOM.render(
<h1>Hello DEVELOPERS!!</h1>,
document.getElementById(‘root’)
);
Step 6: Use the commands listed below to launch your program.
npm start
Or
yarn start
Node.JS
With the help of the JavaScript environment with Node.js, users can execute their code on the server (outside of a browser). With node pack manager or npm, users can download hundreds of free packages, or node modules.
Benefits of using Node.JS
- JavaScript Runtime Environment, open-source
- Adheres to a model that is single-threaded.
- Data Streaming Quickly
- Node.js’s code executes quickly because it is based on the JavaScript Engine of Google Chrome.
- Extremely Flexible
Step 1: In the command window, type the following command to launch a Node.js application. Accept the default configurations.
npm init
Step 2: Make ‘index.js’ the name of the file.
Example
let rectangle= {
perimeter: (x, y)=> (2*(x+y)), area: (x, y)=> (x*y)
};
function Rectangle(l, b) {
console.log(“A rectangle with l = ” +
l + ” and b = ” + b);
if (l <=0 || b <=0) {
console.log(“Error! Rectangle’s length & “
+ “breadth should be greater than 0: l = ”
+ l + “, and b = ” + b);
}
else {
console.log(“Area of the rectangle: ”
+ rectangle.area(l, b));
console.log(“Perimeter of the rectangle: ”
+ rectangle.perimeter(l, b));
}
}
Rectangle(1, 8);
Rectangle(3, 12);
Rectangle(-6, 3);
Step 3: Enter the command below in the command window to launch the node application.
npm start
Understanding of databases: MySQL & MongoDB
MongoDB
Every entry in MongoDB, a NoSQL database, is a document made up of key-value pairs that resemble JSON (JavaScript Object Notation) objects. Because of MongoDB’s flexibility, users can design schemas, databases, tables, and other things.
Benefits of MongoDB for MERN Stack
The following are the advantages of the MERN Stack:
- Faster
- Scalable
- Utilization of JavaScript commands
- Schema Less
- JSON data is saved as strings, values, arrays, objects, and object members.
- Utilizing JSON syntax is quite simple.
- JSON is compatible with a large number of browsers.
- Data Sharing
- Easy Environment Setup
- Flexible Document Model
Step 1: Building a database is merely carried out with a “use” command:
use database_name;
Step 2: Making a table: A new collection or table will be made if the current one doesn’t already exist.
db.createCollection(“collection_name”);
Step 3: Inserting records into the collection.
Db.collection_name.insert
(
{
“id” : 01,
“Name” : “Akil”,
“Department”: “Training”,
“Organization”: “SLA”
}
);
Step 4: Document Querying.
db.collection_name.find({Name : “Akil”}).forEach(printjson);
Conclusion
We hope this MERN Full-Stack developer tutorial is useful for beginners and professionals. Learn them comprehensively in our MERN Full Stack Developer Training in Chennai.